1
Javascript - Variables Mon Mar 12, 2012 9:02 am
Mr.Joker

Mr. WebArtz
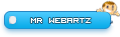
JavaScript - Variables What are variables - With Examples |
Hello everyone. Today I will show you what is variable and how to use it in javascript. Before I go further, you need to know that variable is some word that can storage a value in it. For example you learned in schoold that variable x can be any number. x is variable because it can store a value which is number in Math, but also it can store a characters like words. Lets frist see most simple javascript code:
var - is abbreviations of variable. x - is name of variable. It could be anything else. Any other letter or word. After we name our variable we write that its equal to some value. Its important you understand something. If you want the value be string (words, characters) you will use "" <--- that . But if you want the value of variable be some number you dont need quotation marks. Just a number. Why? Because its an integer, and an integer is writen like that in javascript. Now lets see use of some integer value of variable:
![]()
![]()
1. String + String = String 2. String + Integer = String 4. Integer + Integer = Integer Hope you like my tutorial. |
Notice : This tutorial is copyrighted by WebArtz Forum. You may not publish it on anywhere without written permission from the administrators. |